smtplib and email libraries. This script will send an email with a report as an attachment to a specified list of recipients. Let’s break it down into
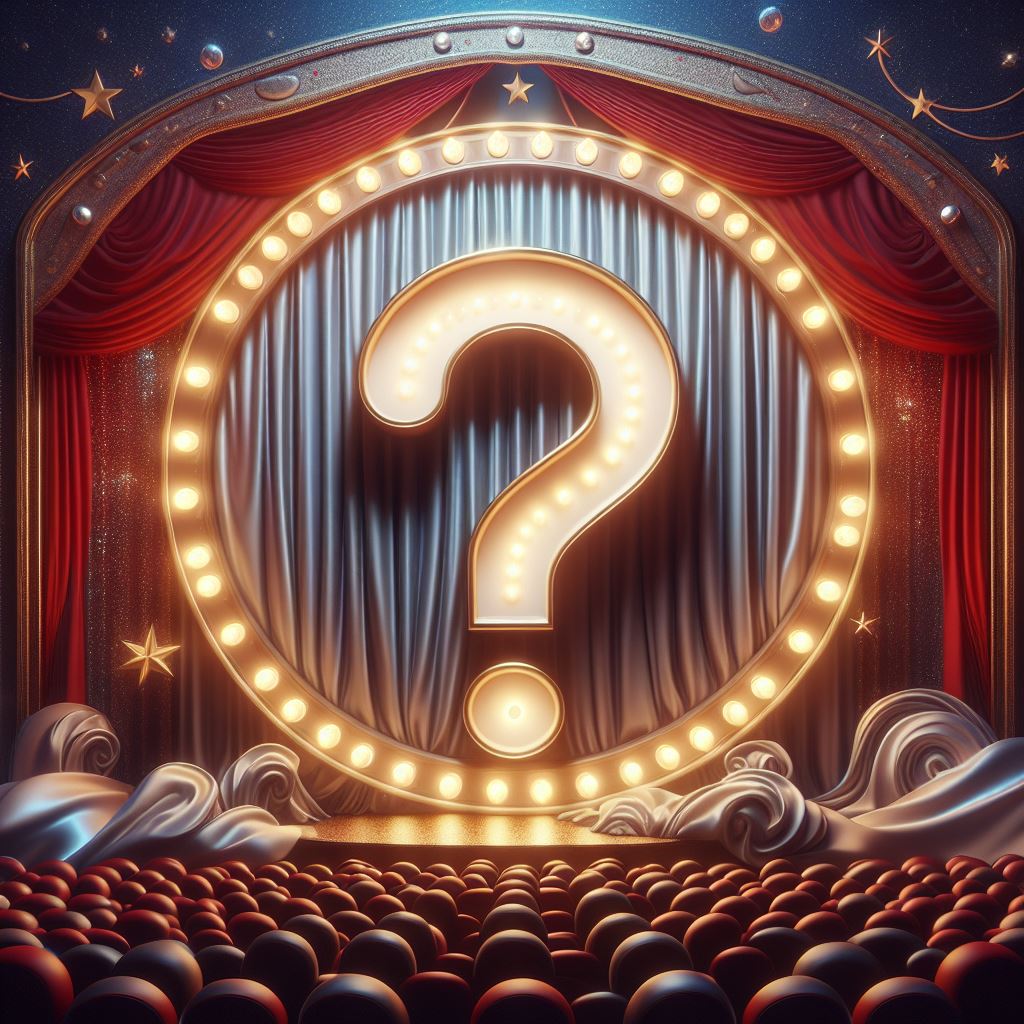
Set up your email credentials: You’ll need an email account to send emails from.
If you’re using Gmail, you’ll also need to allow “less secure apps” to access your account. Alternatively, you can use app-specific passwords for more security.
Write the Python script: This script will generate a report, attach it to an email, and send it.
Set up a task scheduler: You can use cron on Unix-like systems (Linux, macOS) or Task Scheduler on Windows to schedule the script to run daily.
Here’s a sample Python script to get you started:
python
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
from email.mime.base import MIMEBase
from email import encoders
import datetime
def send_email(subject, body, to_email, attachment_filename=None):
from_email = ‘your_email@gmail.com’ Your email address
password = ‘your_password’ Your email password
Create message container
msg = MIMEMultipart()
msg[‘From’] = from_email
msg[‘To’] = ‘, ‘.join(to_email)
msg[‘Subject’] = subject
Add body to email
msg.attach(MIMEText(body, ‘plain’))
Add attachment to email if provided
if attachment_filename:
with open(attachment_filename, ‘rb’) as attachment:
part = MIMEBase(‘application’, ‘octet-stream’)
part.set_payload(attachment.read())
encoders.encode_base64(part)
part.add_header(‘Content-Disposition’, f’attachment; filename= {attachment_filename}’)
msg.attach(part)
Send the email
with smtplib.SMTP(‘smtp.gmail.com’, 587) as server:
server.starttls()
server.login(from_email, password)
server.sendmail(from_email, to_email, msg.as_string())
def generate_report():
Replace this with your report generation logic
today = datetime.date.today()
report = f”Daily report for {today}\n\n”
return report
def main():
subject = ‘Daily Report’
recipients = [‘recipient1@example.com’, ‘recipient2@example.com’] List of recipients
report_body = generate_report()
attachment_filename = ‘report.txt’ Name of the report file
send_email(subject, report_body, recipients, attachment_filename)
if __name__ == “__main__”:
main()
Now, let’s go through how you can set this up:
Set up email credentials: Replace ‘your_email@gmail.com’ with your email address and ‘your_password’ with your email password.
Write the report generation logic: The generate_report() function is a placeholder. Replace it with your actual report generation logic.
Configure recipients and attachment filename: Update the recipients list with the email addresses of the recipients you want to send the report to. Also, update attachment_filename with the name of the report file you want to attach.
Schedule the script: Use cron on Unix-like systems or Task Scheduler on Windows to schedule the script to run daily at the desired time.
For cron, you can add an entry like this to your crontab:
ruby
0 9 /usr/bin/python3 /path/to/your/script.py
This will run the script every day at 9:00 AM.
For Task Scheduler on Windows, you can create a new task and specify the script to run daily at the desired time.
That’s it! With this setup, your Python script will automatically send daily email reports to the specified recipients.